philmasterplus
Active member
Confirmed that as of r20539, JS-based relay scripts are working...almost. Chrome shows the returned HTML source verbatim.
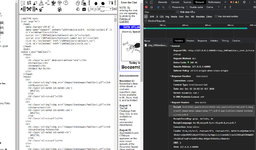
Cursory look at devtools indicates that KoLmafia is returning Fixed in r20550
Two minor issues I've seen so far.
Proxy record fields
Proxy record fields that return other proxy records don't seem to be wrapped properly as JS objects, even in r20539. For example, the following code:
...will throw with:
Also, these "nested" proxy records cannot be compared with regular objects. When tested in the gCLI:
The workaround for this is to manually convert the field to string, then call the get() function:
Importing toString
Attempting to import
...fails with:
It can be trivially avoided by assigning the namespace itself, or by renaming the function:
Since this one is so easy to work around, this is more of a note rather than a request to fix.
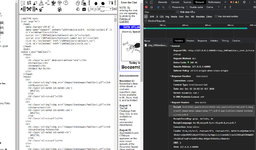
Content-Type: text/javascript
in the HTTP response headers. Special-casing this would be appropriate, since we don't want to break existing cases where script authors serve JS files from within the relay/
directory.Two minor issues I've seen so far.
Proxy record fields
Proxy record fields that return other proxy records don't seem to be wrapped properly as JS objects, even in r20539. For example, the following code:
JavaScript:
const { print } = require("kolmafia");
print(typeof Familiar.get("Angry Goat").hatchling);
...will throw with:
Code:
JavaScript evaluator exception: Invalid JavaScript value of type net.sourceforge.kolmafia.textui.parsetree.Value (file:/C:/Users/Phil/Documents/KoL/scripts/proxy-record-test.js#2)
at file:/C:/Users/Phil/Documents/KoL/scripts/proxy-record-test.js:2
Also, these "nested" proxy records cannot be compared with regular objects. When tested in the gCLI:
Code:
> js Familiar.get("Angry Goat").hatchling === Item.get("goat")
Returned: false
The workaround for this is to manually convert the field to string, then call the get() function:
Code:
> js typeof Item.get(String(Familiar.get("Angry Goat").hatchling)
Returned: object
> js Item.get(String(Familiar.get("Angry Goat").hatchling)) === Item.get("goat")
Returned: true
Importing toString
Attempting to import
toString
from kolmafia
like this...'
JavaScript:
const { toString } = require("kolmafia");
...fails with:
JavaScript error: TypeError: redeclaration of var toString.
It can be trivially avoided by assigning the namespace itself, or by renaming the function:
JavaScript:
const Lib = require("kolmafia"); // Use Lib.toString()
const { toString: formatString } = require("kolmafia");
Since this one is so easy to work around, this is more of a note rather than a request to fix.
Last edited: