You know how, when you do "ash <command>" on the CLI, if you end with a record or an aggregate, mafia will tell you the content of the record/aggregate? Print now does that, always.
1st, this moves the "dump" function that was in AshSingleLineCommand.java and puts it in RuntimeLibrary.java (AS PUBLIC; trying to make sure I get your attention here, Veracity, to be sure you're not against that).
2nd, the two print functions now accept any datatype. If they get sent a string, they print it, as normal, and the function ends. If it's NOT a string, they turn it into a string, and sends it back to them. Then, they look at the datatype of what they initially had, and if it's a composite, they send it to dump() (which now supports colors, too!).
Proof of concept: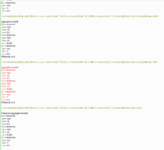
I'm pretty sure there's things that can be improved, such as taking care of the cleaning of the color's string at the start and only passing "that" around, but I'm... currently quite eager to go to sleep, so I'll put this here, let you guys look at it and leave feedback, and if there's anything wrong, I can take care of it tomorrow. G'night (well... it's almost 8Am... :| )
View attachment .patch
1st, this moves the "dump" function that was in AshSingleLineCommand.java and puts it in RuntimeLibrary.java (AS PUBLIC; trying to make sure I get your attention here, Veracity, to be sure you're not against that).
2nd, the two print functions now accept any datatype. If they get sent a string, they print it, as normal, and the function ends. If it's NOT a string, they turn it into a string, and sends it back to them. Then, they look at the datatype of what they initially had, and if it's a composite, they send it to dump() (which now supports colors, too!).
Proof of concept:
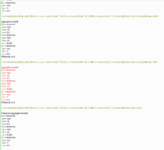
I'm pretty sure there's things that can be improved, such as taking care of the cleaning of the color's string at the start and only passing "that" around, but I'm... currently quite eager to go to sleep, so I'll put this here, let you guys look at it and leave feedback, and if there's anything wrong, I can take care of it tomorrow. G'night (well... it's almost 8Am... :| )
View attachment .patch