Schik
New member
I like the combat bar but using skills and items that you haven't dragged to their own buttons is slower to use - with the old style, I just start typing it and go to the right element in the listbox.
So I made this simple relay script to add a filter to the drop-down combat bar skills & items. At the top of the list, a textbox is added. When you type in it, the list gets filtered to only show things that match your text.
Without a filter:
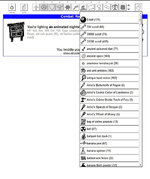
With a filter:
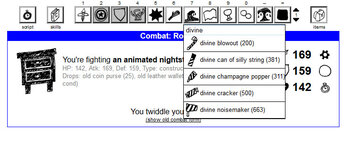
You can grab it via:
It's pretty small but I guess I should document how to use it:
- When you click on either the skill or item button, focus will automatically go to the filter textbox.
- Type stuff in the filter textbox, and the skill/items list will filter as you type.
- Press ESC to quickly clear the textbox (this didn't work in Opera, but does work in IE, Chrome, & FF)
So I made this simple relay script to add a filter to the drop-down combat bar skills & items. At the top of the list, a textbox is added. When you type in it, the list gets filtered to only show things that match your text.
Without a filter:
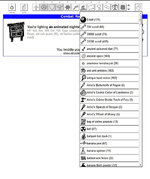
With a filter:
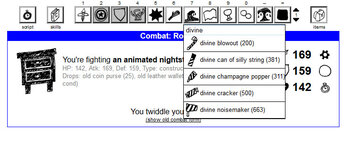
You can grab it via:
Code:
svn checkout https://svn.code.sf.net/p/kolcombatfilter/code/trunk/combatfilter/
It's pretty small but I guess I should document how to use it:
- When you click on either the skill or item button, focus will automatically go to the filter textbox.
- Type stuff in the filter textbox, and the skill/items list will filter as you type.
- Press ESC to quickly clear the textbox (this didn't work in Opera, but does work in IE, Chrome, & FF)
Last edited: